Nota
Haz clic aquí para descargar el código completo del ejemplo o para ejecutar este ejemplo en tu navegador a través de Binder
Aprendizaje en línea de un diccionario de partes de caras¶
Este ejemplo utiliza un gran conjunto de datos de caras para aprender un conjunto de fragmentos de imágenes de 20 x 20 que constituyen caras.
Desde el punto de vista de la programación, es interesante porque muestra cómo utilizar la API en línea de scikit-learn para procesar un conjunto de datos muy grande por porciones. La forma de proceder es que cargamos una imagen a la vez y extraemos aleatoriamente 50 fragmentos de esta imagen. Una vez que hemos acumulado 500 de estos fragmentos (usando 10 imágenes), ejecutamos el método partial_fit
del objeto KMeans en línea, MiniBatchKMeans.
La configuración verbosa de MiniBatchKMeans nos permite ver que algunos conglomerados se reasignan durante las sucesivas invocaciones a partial-fit. Esto se debe a que el número de fragmentos que representan se ha vuelto demasiado bajo, y es mejor elegir un nuevo conglomerado aleatorio.
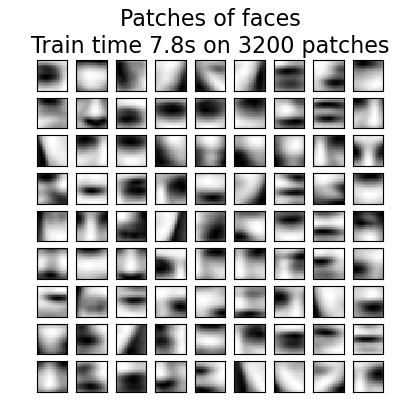
Out:
downloading Olivetti faces from https://ndownloader.figshare.com/files/5976027 to /home/mapologo/scikit_learn_data
Learning the dictionary...
[MiniBatchKMeans] Reassigning 11 cluster centers.
Partial fit of 100 out of 2400
Partial fit of 200 out of 2400
Partial fit of 300 out of 2400
Partial fit of 400 out of 2400
Partial fit of 500 out of 2400
Partial fit of 600 out of 2400
[MiniBatchKMeans] Reassigning 2 cluster centers.
Partial fit of 700 out of 2400
Partial fit of 800 out of 2400
Partial fit of 900 out of 2400
Partial fit of 1000 out of 2400
Partial fit of 1100 out of 2400
Partial fit of 1200 out of 2400
Partial fit of 1300 out of 2400
Partial fit of 1400 out of 2400
Partial fit of 1500 out of 2400
Partial fit of 1600 out of 2400
Partial fit of 1700 out of 2400
Partial fit of 1800 out of 2400
Partial fit of 1900 out of 2400
Partial fit of 2000 out of 2400
Partial fit of 2100 out of 2400
Partial fit of 2200 out of 2400
Partial fit of 2300 out of 2400
Partial fit of 2400 out of 2400
done in 7.82s.
print(__doc__)
import time
import matplotlib.pyplot as plt
import numpy as np
from sklearn import datasets
from sklearn.cluster import MiniBatchKMeans
from sklearn.feature_extraction.image import extract_patches_2d
faces = datasets.fetch_olivetti_faces()
# #############################################################################
# Learn the dictionary of images
print('Learning the dictionary... ')
rng = np.random.RandomState(0)
kmeans = MiniBatchKMeans(n_clusters=81, random_state=rng, verbose=True)
patch_size = (20, 20)
buffer = []
t0 = time.time()
# The online learning part: cycle over the whole dataset 6 times
index = 0
for _ in range(6):
for img in faces.images:
data = extract_patches_2d(img, patch_size, max_patches=50,
random_state=rng)
data = np.reshape(data, (len(data), -1))
buffer.append(data)
index += 1
if index % 10 == 0:
data = np.concatenate(buffer, axis=0)
data -= np.mean(data, axis=0)
data /= np.std(data, axis=0)
kmeans.partial_fit(data)
buffer = []
if index % 100 == 0:
print('Partial fit of %4i out of %i'
% (index, 6 * len(faces.images)))
dt = time.time() - t0
print('done in %.2fs.' % dt)
# #############################################################################
# Plot the results
plt.figure(figsize=(4.2, 4))
for i, patch in enumerate(kmeans.cluster_centers_):
plt.subplot(9, 9, i + 1)
plt.imshow(patch.reshape(patch_size), cmap=plt.cm.gray,
interpolation='nearest')
plt.xticks(())
plt.yticks(())
plt.suptitle('Patches of faces\nTrain time %.1fs on %d patches' %
(dt, 8 * len(faces.images)), fontsize=16)
plt.subplots_adjust(0.08, 0.02, 0.92, 0.85, 0.08, 0.23)
plt.show()
Tiempo total de ejecución del script: (0 minutos 32.656 segundos)